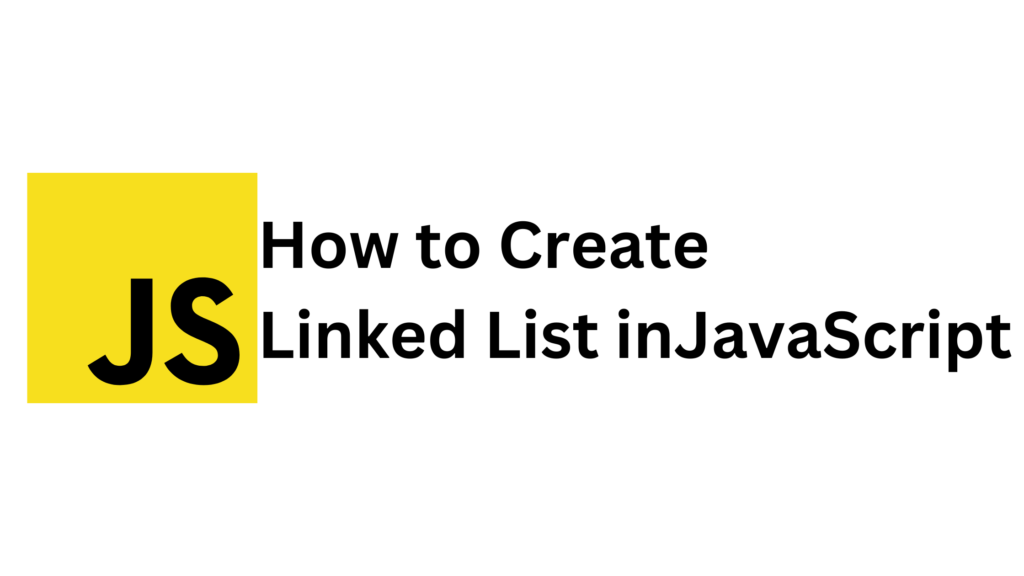
In this blog we are going to learn how to create a Linked List in JavaScript
But What is Linked List?
A linked list is a linear data structure where elements are connected to each other through pointers. Each element in the linked list, called a node, contains a value and a reference to the next node in the list
class Node {
constructor(value, next = null) {
this.value = value;
this.next = next;
}
}
class LinkedList {
constructor() {
this.head = null;
this.tail = null;
}
add(value) {
const node = new Node(value);
if (!this.head) {
this.head = node;
this.tail = node;
return;
}
this.tail.next = node;
this.tail = node;
}
*[Symbol.iterator]() {
let node = this.head;
while (node) {
yield node.value;
node = node.next;
}
}
}
const list = new LinkedList();
list.add(1);
list.add(2);
list.add(3);
for (const value of list) {
console.log(value);
}
In this example, the Node
class represents a node in the linked list, and the LinkedList
class represents the linked list itself. The LinkedList
class has a head
property that points to the first node in the list, and a tail
property that points to the last node in the list. The add
method creates a new node with the given value and adds it to the end of the list. The linked list is made iterable by implementing the Symbol.iterator
method, which allows it to be used in a for...of
loop.