Are you interested in creating interactive animations for your web projects? HTML5 canvas provides a powerful tool for rendering graphics and animations directly in the browser. In this tutorial, we’ll explore the basics of creating a simple animated ball using HTML5 canvas and JavaScript.
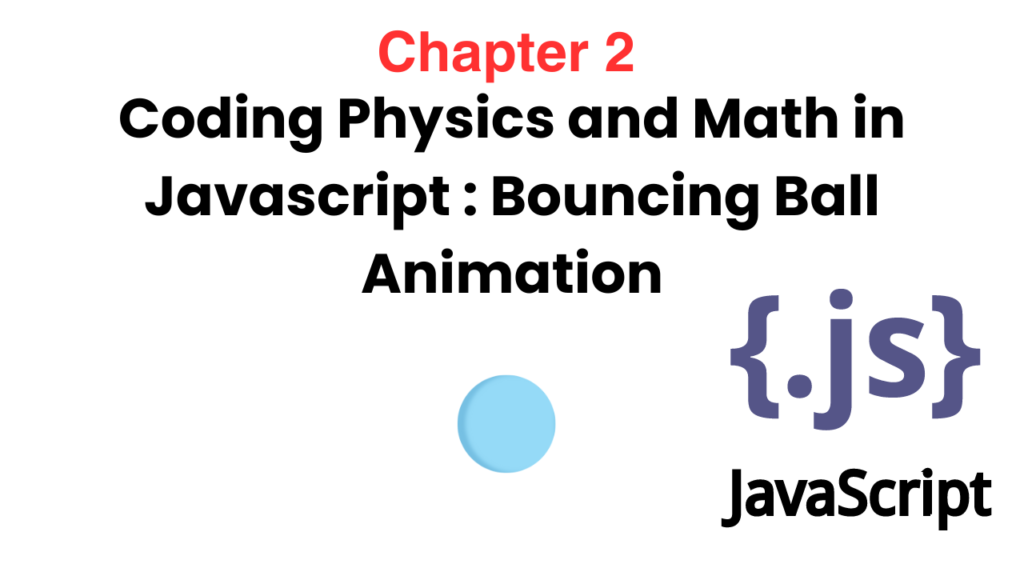
Setting Up the HTML File
Let’s start by creating a basic HTML file that includes a canvas element where our animation will be displayed.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Canvas Animation</title>
</head>
<body>
<canvas id="canvas"></canvas>
<script src="script.js"></script>
</body>
</html>
Creating the JavaScript Animation
Next, let’s create a JavaScript file (script.js
) where we’ll write the code for our animation. This script will handle the animation logic, such as updating the position of the ball and redrawing it on the canvas.
const canvas = document.getElementById('canvas');
const ctx = canvas.getContext('2d')
// Set canvas size to match window size
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
let x = canvas.width/2;
let y = canvas.height/2;
let dx = 2;
let dy = -2;
let radius = 30;
function drawBall(){
ctx.clearRect(0,0,canvas.width,canvas.height);
ctx.beginPath();
ctx.arc(x,y,radius,0,Math.PI*2);
ctx.fillStyle="#0095DD"
ctx.fill();
ctx.closePath();
}
function update(){
drawBall();
if(x+dx >canvas.width-radius || x+dx<radius){
dx = -dx
}
if(y+dy>canvas.height-radius || y+dy<radius){
dy= -dy
}
x+=dx;
y+=dy;
}
// Update the animation every 10 milliseconds
setInterval(update,10);
Setting Up the Canvas
To begin, we need to set up our canvas element and get its 2D rendering context:
const canvas = document.getElementById('canvas');
const ctx = canvas.getContext('2d');
We also set the canvas size to match the window size to ensure that our animation covers the entire viewport:
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
Defining Ball Properties
Next, we define variables to represent the position, velocity, and size of the ball:
let x = canvas.width / 2;
let y = canvas.height / 2;
let dx = 2;
let dy = -2;
let radius = 30;
Here, x
and y
represent the coordinates of the center of the ball, dx
and dy
represent the change in position (velocity) in the x and y directions, and radius
represents the radius of the ball.
Drawing the Ball
The drawBall
function is responsible for clearing the canvas and drawing the ball at its current position:
function drawBall() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.beginPath();
ctx.arc(x, y, radius, 0, Math.PI * 2);
ctx.fillStyle = "#0095DD";
ctx.fill();
ctx.closePath();
}
Updating the Animation
The update
function is called repeatedly using setInterval
to update the position of the ball and handle collisions with the canvas boundaries:
function update() {
x += dx;
y += dy;
if (x + dx > canvas.width - radius || x + dx < radius) {
dx = -dx;
}
if (y + dy > canvas.height - radius || y + dy < radius) {
dy = -dy;
}
drawBall();
}
setInterval(update, 10);
Conclusion
In this blog post, we’ve explored a simple example of animating a ball using HTML5 canvas and JavaScript. By understanding the basics of canvas drawing and animation, you can create a wide range of dynamic and interactive graphics for your web projects. Experiment with different shapes, colors, and motion patterns to create unique and engaging animations!