Palindromic strings are fascinating patterns that read the same forwards and backward. In this TypeScript tutorial, we’ll explore how to find all possible palindromic strings from a given input string. We’ll walk through the process step by step, providing a clear and detailed explanation along the way.
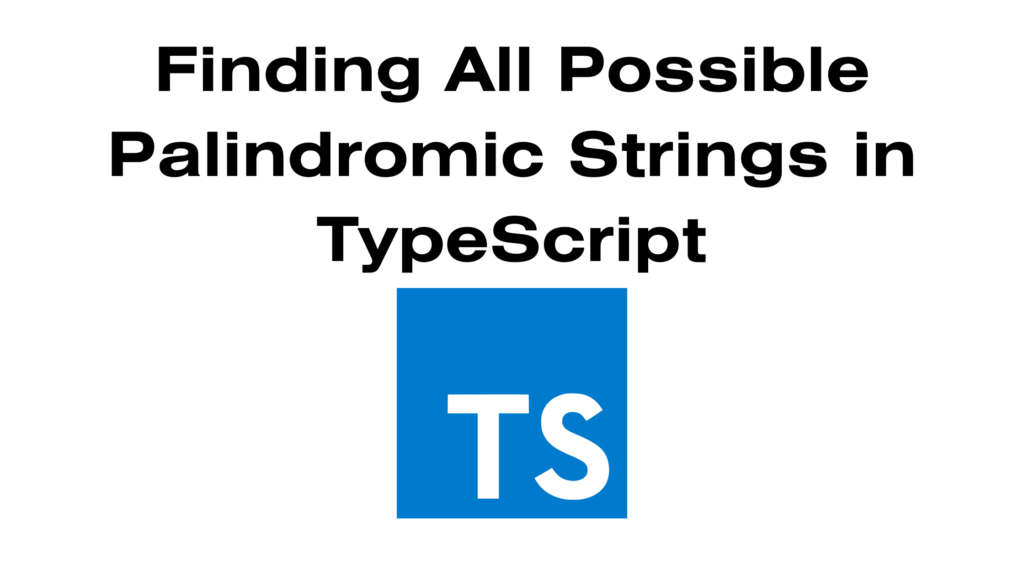
Prerequisites
Before we start, make sure you have the following:
- Node.js and npm installed on your system.
- A code editor (e.g., Visual Studio Code) for writing TypeScript.
Setting Up the TypeScript Project
- Create a New Directory:
Start by creating a new directory for your project. Open your terminal and run:
mkdir palindrome-finder
cd palindrome-finder
- Initialize a TypeScript Project:
Inside your project directory, initialize a TypeScript project:
npm init -y
npm install typescript --save-dev
- Create a TypeScript Configuration File:
Create atsconfig.json
file in your project directory to configure TypeScript:
{
"compilerOptions": {
"target": "ES6",
"outDir": "./dist",
"rootDir": "./src",
"module": "commonjs"
}
}
- Create a
src
Directory:
Create asrc
directory where your TypeScript files will reside:
mkdir src
- Create the TypeScript File:
Inside thesrc
directory, create a TypeScript file namedpalindrome.ts
. This is where we’ll write our code.
Writing the TypeScript Code
Now, let’s write the TypeScript code to find all possible palindromic strings from an input string.
// src/palindrome.ts
function isPalindrome(str: string): boolean {
const cleanStr = str.replace(/[^a-zA-Z0-9]/g, '').toLowerCase();
const reversedStr = cleanStr.split('').reverse().join('');
return cleanStr === reversedStr;
}
function getAllPalindromes(input: string): string[] {
const palindromes: string[] = [];
const length = input.length;
for (let i = 0; i < length; i++) {
for (let j = i + 1; j <= length; j++) {
const subStr = input.substring(i, j);
if (isPalindrome(subStr)) {
palindromes.push(subStr);
}
}
}
return palindromes;
}
// Example usage
const inputString = "racecar";
const palindromes = getAllPalindromes(inputString);
console.log("All Palindromes in the inputString:", palindromes);
In this code:
isPalindrome
is a function that checks whether a given string is a palindrome or not. It removes non-alphanumeric characters and spaces, converts the string to lowercase, and compares it to its reverse.getAllPalindromes
is the main function that finds all palindromic substrings in the input string. It iterates through all possible substrings using two nested loops and checks each substring for palindromicity.- We provide an example usage at the end of the code, demonstrating how to find all palindromes in the
inputString
.
Running the TypeScript Code
- Compile the TypeScript Code:
To compile the TypeScript code into JavaScript, run the following command in your project directory:
npx tsc
- Run the JavaScript Code:
After compiling, you will find the compiled JavaScript file in thedist
directory. You can run it using Node.js:
node dist/palindrome.js
Conclusion
In this TypeScript tutorial, we’ve learned how to find all possible palindromic strings within an input string. We’ve created a TypeScript project, written the necessary code, and executed it to find palindromic substrings. This knowledge can be useful in various applications, from text processing to algorithmic problem-solving.
Feel free to modify the code to suit your specific needs or integrate it into your own TypeScript projects. Happy coding!