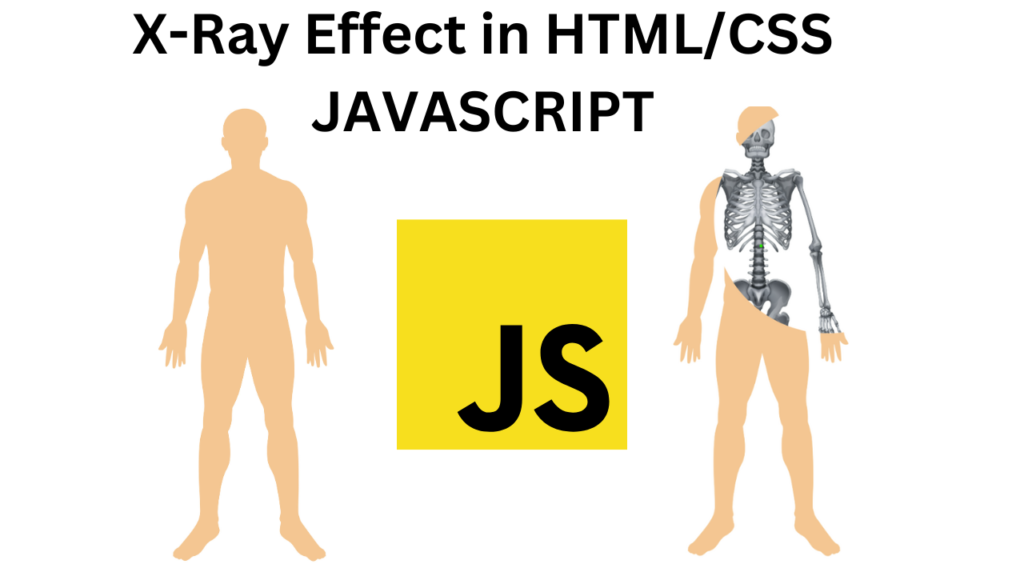
Introduction:
SVG (Scalable Vector Graphics) is a powerful tool for creating interactive and visually appealing web graphics. In this blog post, we’ll explore how to create a dynamic SVG mask effect using JavaScript. This effect will allow an image to reveal its underlying content as the mouse cursor moves across it. We’ll break down the code step by step and explain how it works.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<svg width="250" height="650">
<image xlink:href="human.jpg" width="250" height="600"/>
<clipPath id="XrayMask">
<circle id="maskCircle" cx="50%" cy="50%" r="10%"/>
</clipPath>
<image clip-path="url(#XrayMask)"
xlink:href="skeleton.jpg" width="250" height="600"/>
</svg>
<script>
let animate = document.getElementById("maskCircle");
window.addEventListener('mousemove',function(e){
animate.setAttribute("cx",e.pageX)
animate.setAttribute("cy",e.pageY)
},false)
</script>
</body>
</html>
The SVG Element:
- We begin with an SVG element, which is a vector graphic format that allows us to create two images stacked on top of each other.
- The first
<image>
element displays an image named “human.jpg” and sets its dimensions to 250 pixels in width and 600 pixels in height. - Next, we define a
<clipPath>
element with the id “XrayMask”. This element will serve as the mask for our image effect. - Inside the
<clipPath>
, we place a<circle>
element with the id “maskCircle”. This circle will be the moving part of our mask. - The second
<image>
element displays an image named “skeleton.jpg” and is subject to the masking effect defined by the<clipPath>
.
The JavaScript:
- We select the “maskCircle” element using
document.getElementById("maskCircle")
and store it in theanimate
variable. - We add a
mousemove
event listener to thewindow
object. This listener triggers a function whenever the mouse cursor is moved. - Inside the event listener function, we use
animate.setAttribute("cx", e.pageX)
andanimate.setAttribute("cy", e.pageY)
to update the position of the “maskCircle” element based on the mouse cursor’s coordinates. This creates the dynamic masking effect.