In this blog we are going to learn how to make Cicrular Slider in react
First you need to install dependencies
npm i @fseehawer/react-circular-slider
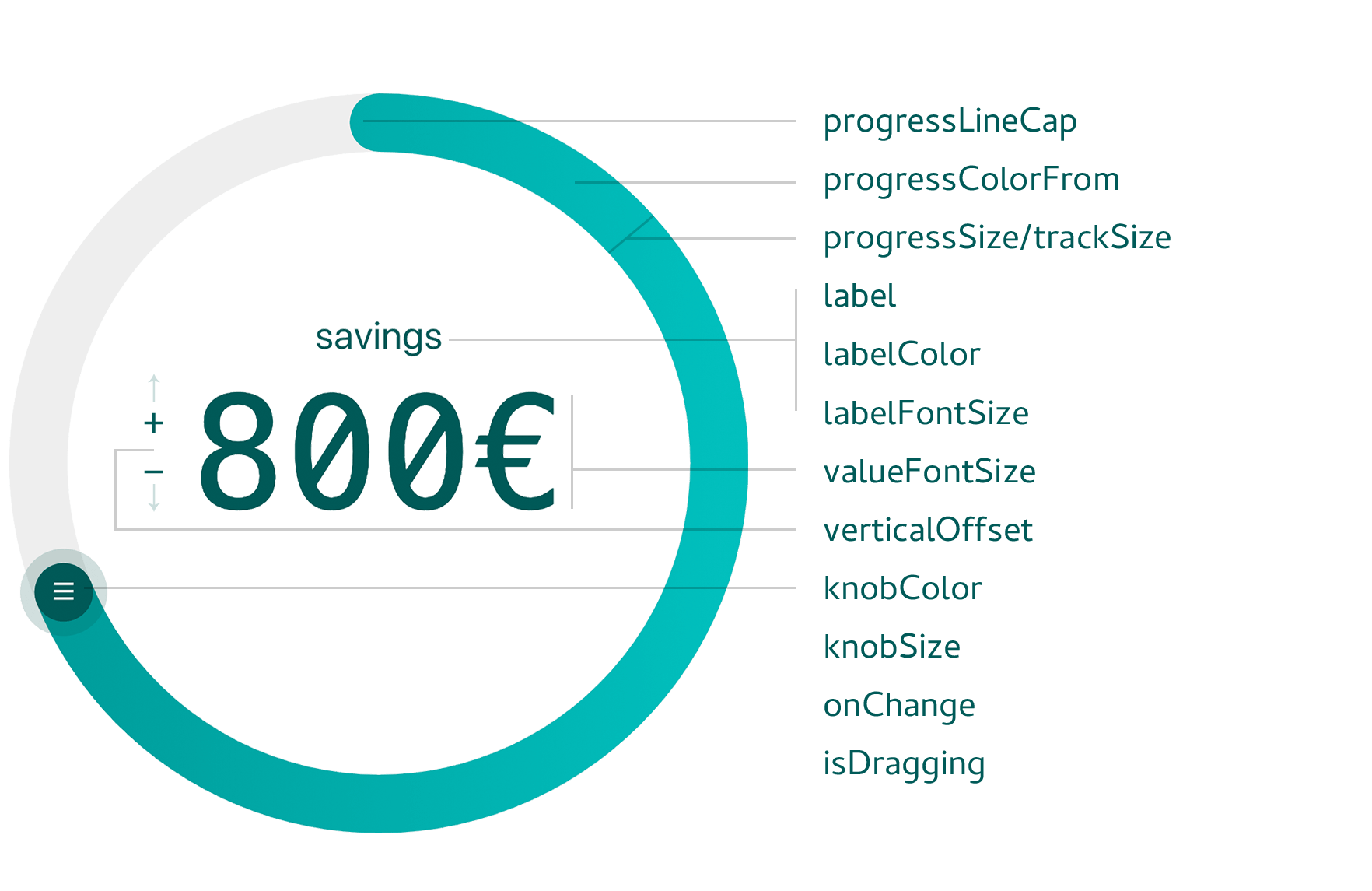
Code Use in Tutorial
import React from “react”;
import CircularSlider from “@fseehawer/react-circular-slider”;
import { GoDashboard } from “react-icons/go”;
import “./App.css”;
const CircularSliderExample = () => {
const [value, setValue] = React.useState(0);
return (
<div className=”app”>
<CircularSlider
width={200}
height={200}
min={0}
max={20}
label=”Mechanica Coder”
dataIndex={value}
onChange={(value) => setValue(value)}
>
<GoDashboard x=”9″ y=”9″ width=”50px” height=”50px” />
</CircularSlider>
</div>
);
};
export default CircularSliderExample;
Props
prop | type | default | Affects |
---|---|---|---|
width | number | 280 | width of the slider |
direction | number | 1 | clockwise (1) or anticlockwise (-1) |
min | number | 0 | smallest value |
max | number | 360 | largest value |
data | array | [] | array of data to be spread in 360° |
dataIndex | number | 0 | initially place knob at a certain value in the array |
knobColor | string | #4e63ea | knob color |
knobSize | number | 32 | knob size |
hideKnob | boolean | false | hide knob |
knobDraggable | boolean | true | knob draggable |
knobPosition | string | top | knob’s 0 position to be top, right, bottom or left |
label | string | ANGLE | label |
labelColor | string | #272b77 | label and value color |
labelBottom | boolean | false | label position at bottom |
labelFontSize | string | 1rem | label font-size |
valueFontSize | string | 4rem | label value font-size |
appendToValue | string | ” | append character to value |
prependToValue | string | ” | prepend character to value |
renderLabelValue | jsx | null | add custom jsx code for the labels and styles |
verticalOffset | string | 2rem | vertical offset of the label and value |
hideLabelValue | boolean | false | hide label and value |
progressColorFrom | string | #80C3F3 | progress track gradient start color |
progressColorTo | string | #4990E2 | progress track gradient end color |
progressSize | number | 8 | progress track size |
progressLineCap | string | round | progress track cap to be round or flat |
trackColor | string | #DDDEFB | background track color |
trackSize | number | 8 | background track size |
onChange | func | value => {} | returns label value |
isDragging | func | value => {} | returns isDragging value |
Some Other Example of the code Work
You can find it here