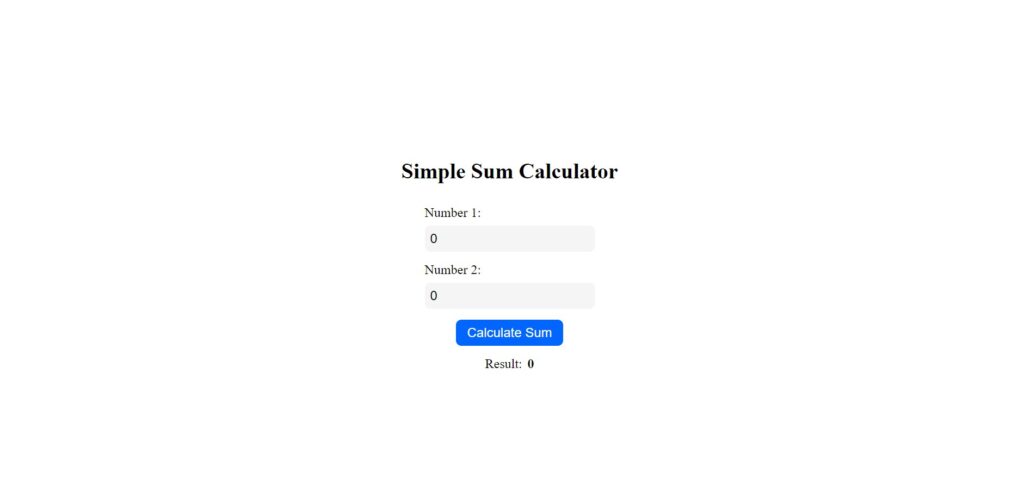
In this app, we’re using the useState
hook to keep track of the two input values and the result of the sum. We have an onChange
handler for each input that updates its corresponding state variable whenever the user types in a new value. When the user clicks the “Calculate Sum” button, we call the handleSum
function, which sets the result
state variable to the sum of the two input values. Finally, we display the result below the button using a span
element.
Note: We are using Function Base Component to Make this project
import React, { useState } from "react";
import "./styles.css";
function App() {
const [num1, setNum1] = useState(0);
const [num2, setNum2] = useState(0);
const [result, setResult] = useState(0);
const handleSum = () => {
setResult(num1 + num2);
};
return (
<div className="container">
<h1>Simple Sum Calculator</h1>
<div className="input-container">
<label>Number 1:</label>
<input
type="number"
value={num1}
onChange={(e) => setNum1(parseInt(e.target.value))}
/>
</div>
<div className="input-container">
<label>Number 2:</label>
<input
type="number"
value={num2}
onChange={(e) => setNum2(parseInt(e.target.value))}
/>
</div>
<button className="calc-button" onClick={handleSum}>
Calculate Sum
</button>
<div className="result-container">
<label>Result:</label>
<span>{result}</span>
</div>
</div>
);
}
export default App;
.container {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
height: 100vh;
}
h1 {
font-size: 2rem;
margin-bottom: 2rem;
}
.input-container {
display: flex;
flex-direction: column;
margin-bottom: 1rem;
}
label {
font-size: 1.2rem;
margin-bottom: 0.5rem;
}
input {
font-size: 1.2rem;
padding: 0.5rem;
border-radius: 0.5rem;
border: none;
background-color: #f5f5f5;
}
.calc-button {
font-size: 1.2rem;
padding: 0.5rem 1rem;
border-radius: 0.5rem;
border: none;
background-color: #0066ff;
color: #fff;
cursor: pointer;
transition: all 0.2s ease-in-out;
}
.calc-button:hover {
transform: scale(1.1);
}
.result-container {
font-size: 1.2rem;
margin-top: 1rem;
}
span {
font-weight: bold;
margin-left: 0.5rem;
}