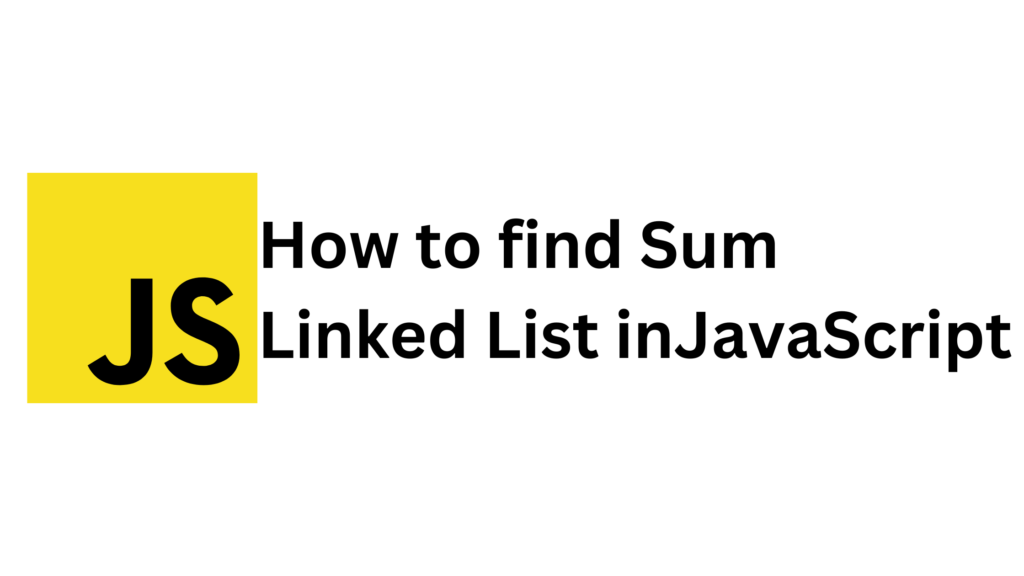
In this blog we are going to learn how to find sum of linkedlist in javascript
class Node {
constructor(value, next = null) {
this.value = value;
this.next = next;
}
}
class LinkedList {
constructor() {
this.head = null;
this.tail = null;
}
add(value) {
const node = new Node(value);
if (!this.head) {
this.head = node;
this.tail = node;
return;
}
this.tail.next = node;
this.tail = node;
}
sum() {
let sum = 0;
let node = this.head;
while (node) {
sum += node.value;
node = node.next;
}
return sum;
}
}
const list = new LinkedList();
list.add(1);
list.add(2);
list.add(3);
console.log(list.sum()); // 6