Are you looking to enhance your website’s user experience? A visually appealing and animated progress bar can provide users with real-time feedback and make your site more interactive. In this tutorial, we’ll show you how to create a stylish progress bar using HTML, CSS, and JavaScript.
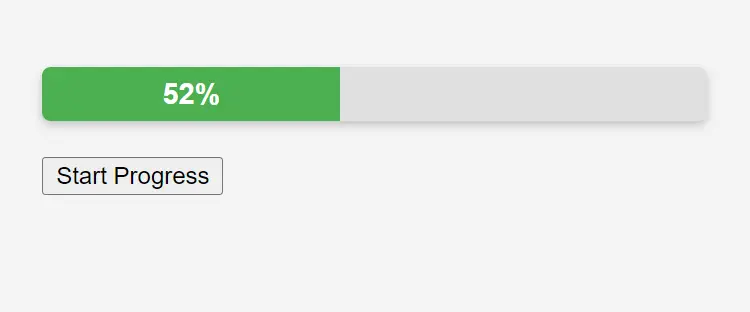
What You’ll Learn
- How to structure a progress bar with HTML
- Styling the progress bar with CSS for better aesthetics
- Adding animation using JavaScript
- Tips for optimizing your blog post for SEO
Step 1: Setting Up the HTML Structure
Let’s start by creating a simple HTML structure for our progress bar. Open your WordPress editor and add the following code:
htmlCopy code<div class="progress-container">
<div class="progress-bar" id="progress-bar">
<span id="progress-text">0%</span>
</div>
</div>
<button onclick="startProgress()">Start Progress</button>
Explanation
div.progress-container
: This is the outer container that holds the progress bar.div.progress-bar
: This inner div represents the animated progress.button
: A button to trigger the animation.
Step 2: Styling the Progress Bar with CSS
Next, let’s add some CSS to make our progress bar visually appealing. You can place this CSS in the custom CSS section of your WordPress theme or in the stylesheet.
cssCopy codebody {
font-family: Arial, sans-serif;
background-color: #f4f4f4;
padding: 20px;
}
.progress-container {
width: 100%;
background-color: #e0e0e0;
border-radius: 5px;
overflow: hidden;
height: 30px;
margin: 20px 0;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.2);
}
.progress-bar {
height: 100%;
width: 0;
background-color: #4caf50; /* Green color */
transition: width 0.4s ease;
position: relative;
}
#progress-text {
position: absolute;
color: white;
left: 50%;
transform: translateX(-50%);
font-weight: bold;
font-size: 16px; /* Increase font size */
line-height: 30px; /* Center text vertically */
}
Explanation
- The background color and box shadow provide a modern look.
- The text is styled to be bold and centered for better readability.
Step 3: Adding JavaScript for Animation
Now, let’s add JavaScript to handle the animation of the progress bar. Insert the following script before the closing </body>
tag in your HTML.
htmlCopy code<script>
function startProgress() {
const progressBar = document.getElementById('progress-bar');
const progressText = document.getElementById('progress-text');
let width = 0;
const interval = setInterval(() => {
if (width >= 100) {
clearInterval(interval);
} else {
width++;
progressBar.style.width = width + '%';
progressText.innerText = width + '%'; // Update the percentage text
}
}, 50);
}
</script>
Explanation
- The
startProgress
function increments the width of the progress bar every 50 milliseconds, updating the displayed percentage.